For Developers: How to interact with Bitfinity Smart Contracts from the Front-end
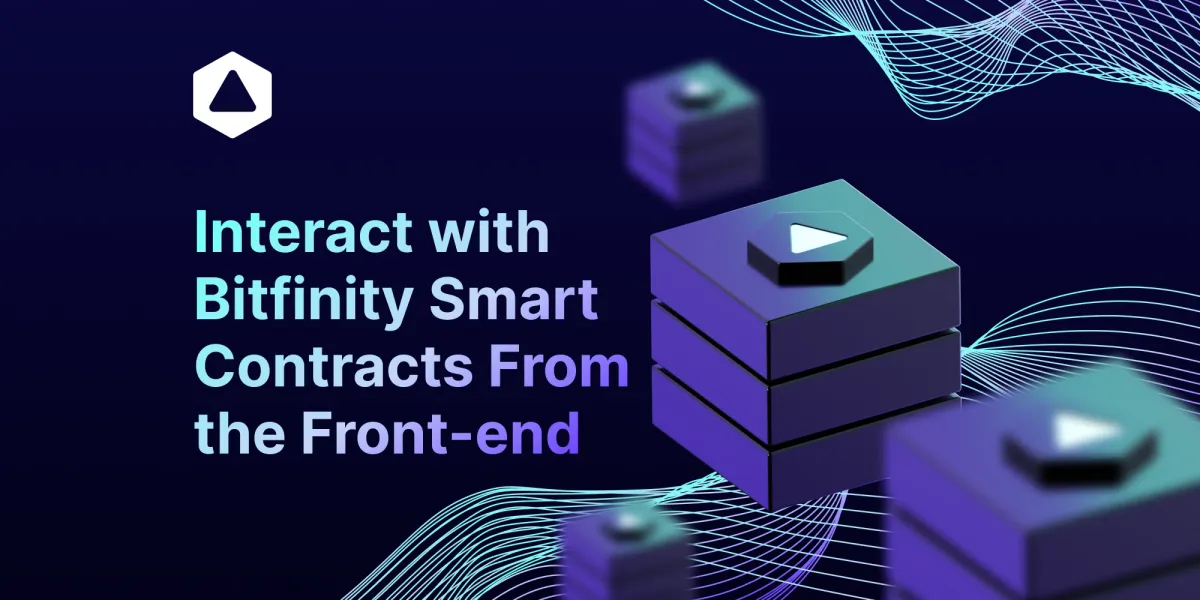
Interacting with smart contracts deployed on the Bitfinity blockchain from a frontend typically involves using a web3 library, such as Web3.js, to communicate with the blockchain network. There are also other libraries you could use like wagmi.sh. Wagmi is a collection of React Hooks containing everything you need to start working with Ethereum. wagmi makes it easy to "Connect Wallet," display ENS and balance information, sign messages, interact with contracts, and much more — all with caching, request deduplication, and persistence so this can be for react applications.
Setup Development Environment
In this guide, we will be using Reactjs. You can follow how to do this using the this guide https://legacy.reactjs.org/docs/create-a-new-react-app.html
Install Dependency Web3 in the root of the project
npm install web3
Once we have that installed, we need to check if Metamask is installed and enabled. For the sake of this tutorial we can work in app.js but you can manipulate your files any how you want or create react hooks or reusable functions to make your work easy.
import Web3 from "web3";
import { useState } from "react";
function App() {
const [web3, setWeb3] = useState(null)
const connectToWallet = async () => {
if (typeof window.ethereum !== "undefined") {
const web3Instance = new Web3(window.ethereum);
try {
await window.ethereum.enable();
setWeb3(web3Instance);
} catch (error) {
console.error("Access to MetaMask account denied");
}
} else {
console.error("Please install Metamask extension");
}
};
return (
<div className="App">
<button onClick={() => connectToWallet()}>Connect to Wallet</button>
</div>
);
}
export default App;
Let’s connect to the smart contract
Retrieve the compiled contract ABI (Application Binary Interface) and bytecode from the Bitfinity blockchain or the contract developer. example can be found here https://github.com/infinity-swap/bitfinity-examples-nft-marketplace/blob/main/utils/MyNFT.json . You can read more about ABIs here https://www.quicknode.com/guides/ethereum-development/smart-contracts/what-is-an-abi
Update the code above by add this
import Web3 from "web3";
import nftABI from "./abi.json";
const { abi: contractABI } = nftABI;
import Web3 from "web3";
import nftABI from "./abi.json";
import { useState } from "react";
const { abi: contractABI } = nftABI;
const contractAddress = "0x14419361611f6c8eAf83957747427d76c18F59cd"; // example Address of the deployed smart contract
function App() {
const [web3, setWeb3] = useState(null);
const [contract, setContract] = useState(null);
const connectToWallet = async () => {
if (typeof window.ethereum !== "undefined") {
const web3Instance = new Web3(window.ethereum);
try {
await window.ethereum.enable();
setWeb3(web3Instance);
const contractInstance = new web3Instance.eth.Contract(
contractABI,
contractAddress
);
setContract(contractInstance);
} catch (error) {
console.error("Access to MetaMask account denied");
}
} else {
console.error("Please install Metamask extension");
}
};
Let’s create an instance of the contract and add read and write functions.
For this, you will need the contract address which you can get from the developer. You can also reference what the contract address is and how to get this from https://www.blog.bitfinity.network/how-to-deploy-a-solidity-smart-contract-to-icp-using-the-bitfinity-evm/
import Web3 from "web3";
import nftABI from "./abi.json";
import { useState } from "react";
const { abi: contractABI } = nftABI; // ABI json
const contractAddress = "0x14419361611f6c8eAf83957747427d76c18F59cd"; // example Address of the deployed smart contract
function App() {
const [web3, setWeb3] = useState(null); // web3 instance
const [contract, setContract] = useState(null);// contract instance
//connect and create contract instance
const connectToWallet = async () => {
if (typeof window.ethereum !== "undefined") {
const web3Instance = new Web3(window.ethereum);
try {
await window.ethereum.enable();
setWeb3(web3Instance);
const contractInstance = new web3Instance.eth.Contract(
contractABI,
contractAddress
);
setContract(contractInstance);
} catch (error) {
console.error("Access to MetaMask account denied");
}
} else {
console.error("Please install Metamask extension");
}
};
// replace someMethod() with the method you want to call
const readContract = () => {
contract.methods.someMethod().call((error, result) => {
if (error) {
console.error(error);
} else {
console.log(result);
}
});
};
const writeContract = () => {
const accounts = await web3.eth.getAccounts(); // The selected Metamask account
const account = accounts[0];
const arg1 = 0;
const arg2 = 0;
const data = contract.methods.someMethod(arg1, arg2).encodeABI();
web3.eth
.getTransactionCount(account)
.then((nonce) => {
const transactionObject = {
to: contractAddress,
gas: 200000, // Adjust the gas limit accordingly
data,
nonce,
chainId: 1, // Replace with the correct chain ID of Bitfinity
};
return window.ethereum.request({
method: "eth_sendTransaction",
params: [transactionObject],
});
})
.then((transactionHash) => {
console.log("Transaction sent:", transactionHash);
})
.catch((error) => {
console.error(error);
});
};
return (
<div className="App">
<button onClick={() => connectToWallet()}>Connect to Wallet</button>
</div>
);
}
export default App;
Let’s write another function to make transfer to a contract. Replace makePayment() with the appropriate function name.
const makePayment = async () => {
const accounts = await web3.eth.getAccounts(); // The selected Metamask account
const account = accounts[0];
if (!contract || !account) {
console.error('Contract or account not initialized');
return;
}
const paymentAmount = web3.utils.toWei('0.1', 'ether'); // Specify the payment amount in Ether
try {
await contract.methods.makePayment().send({ from: account, value: paymentAmount });
console.log('Payment successful');
} catch (error) {
console.error('Payment failed:', error);
}
};
With this information I believe you can interact with your smart contracts. There are other ways of getting this done as i have started earlier. You can also take a look at this article https://www.web3.university/tracks/create-a-smart-contract/integrate-your-smart-contract-with-a-frontend which also explains how to interact with Smart Contract with the help of Alchemy. The idea is the same so whether you are using Vuejs, Reactjs or any other frontend framework, you can apply the same logic to create the same outcome. You can also check out wagmi’s examples which uses react hooks to interact with smart contracts. It is very easy to interact into your work. https://wagmi.sh/examples/connect-wallet
Where to go from here?
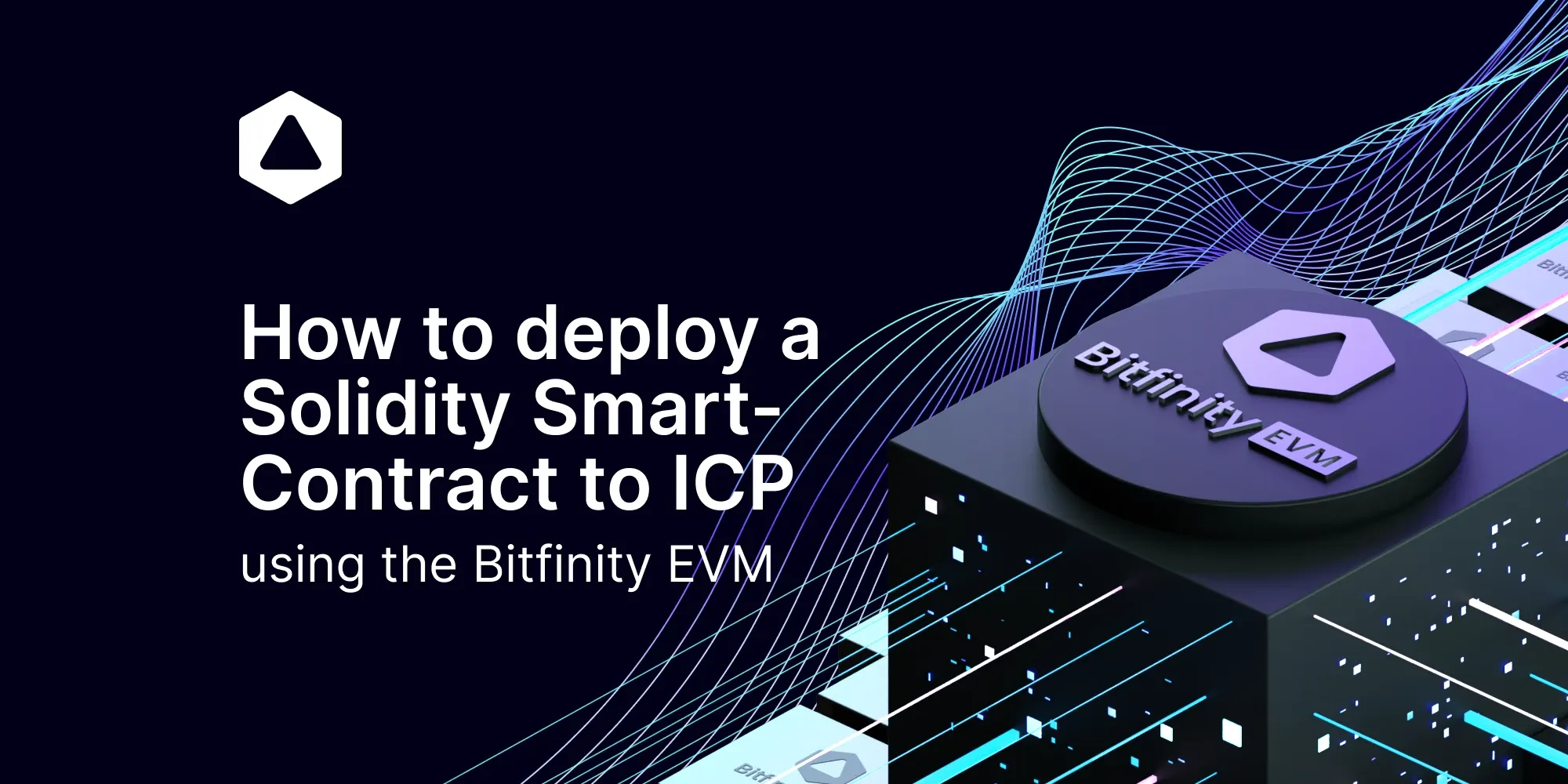
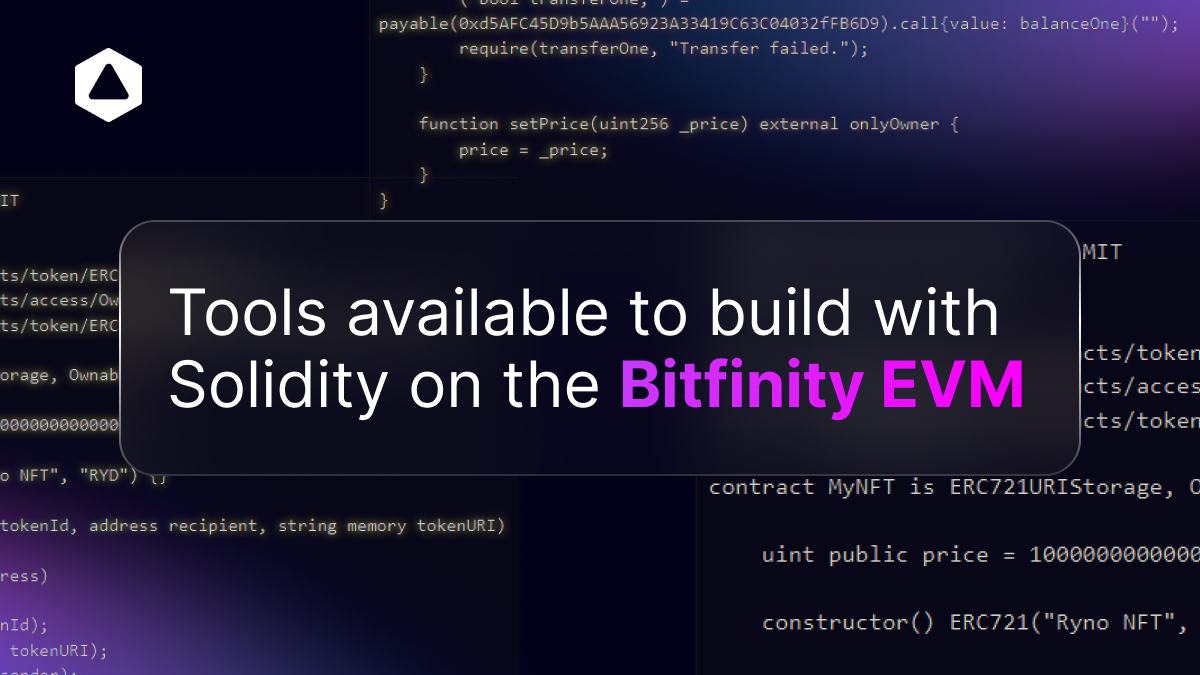
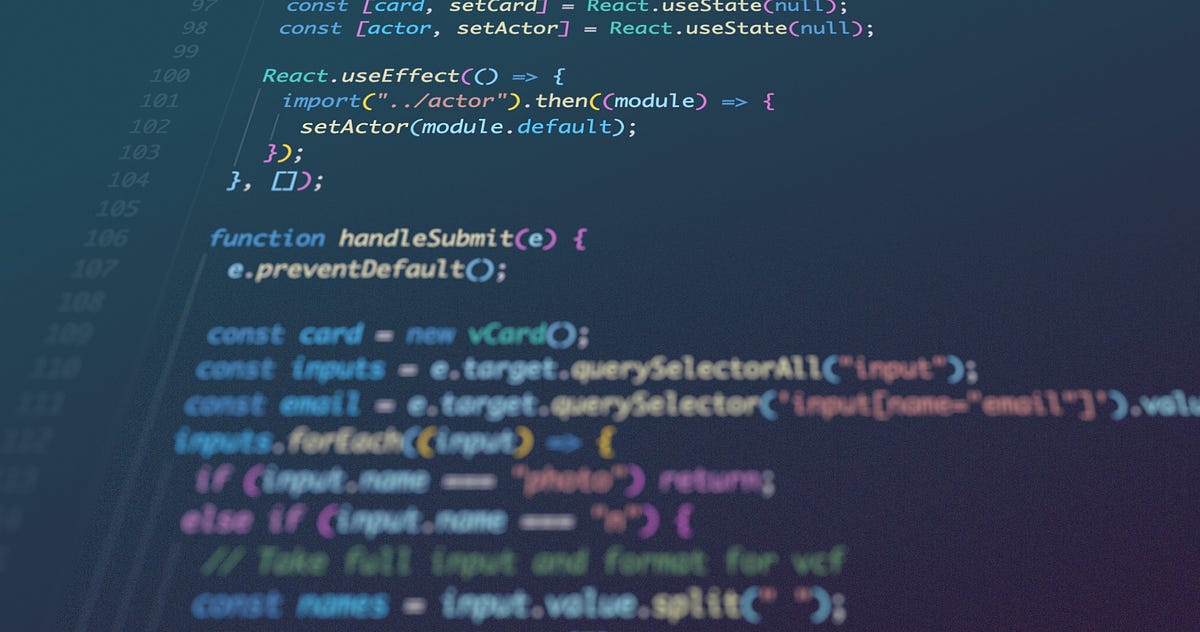
Keep on Building.
Bitfinity Wallet | Bitfinity Network | Twitter | Telegram | Discord | Github
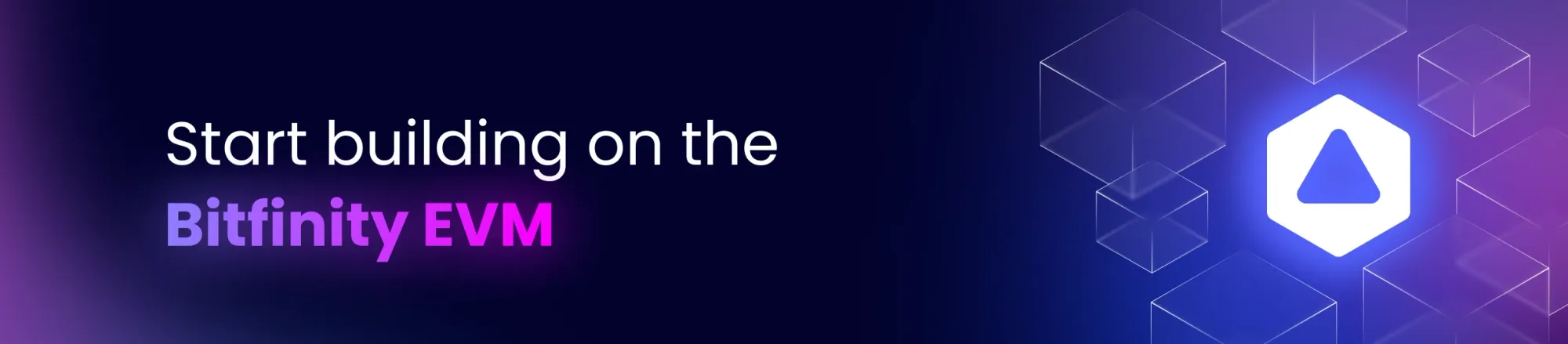
Comments ()