Solidity Contract Optimizations: How to minimise network costs and strengthen security
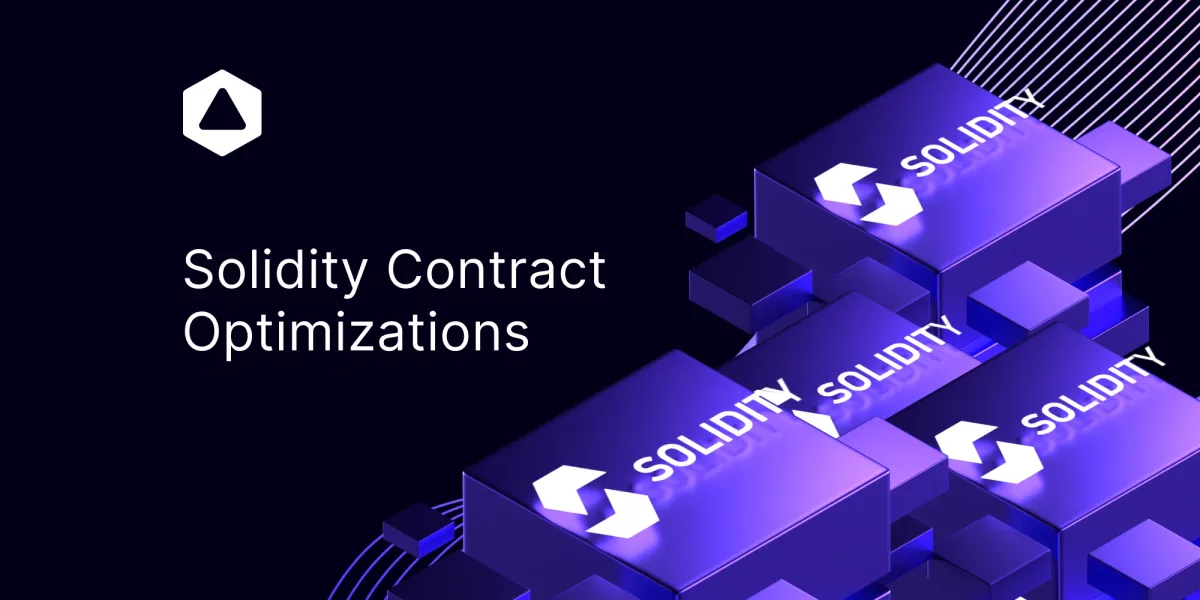
As the popularity of smart contracts continues to grow, the associated transaction costs and security concerns have become crucial points of focus.
Luckily, Ethereum Virtual Machines such as the Bitfinity EVM can execute Solidity contracts at considerably lower costs to users and developers as compared to Ethereum Mainnet. However it's still important to explore optimisation techniques.
In this article, we will shed light on the various optimization techniques that can be employed to minimize transaction costs and enhance the security of smart contracts.
Understanding Smart Contract Execution and Transaction Costs
Before diving into the optimization techniques, it is essential to understand the underlying mechanics of smart contract execution and the factors contributing to transaction costs. Smart contracts are written in programming languages specifically designed for blockchain platforms, such as Solidity for Ethereum.
They are deployed on the blockchain and executed by the network nodes (network nodes are computers that participate in the blockchain network. They validate and execute smart contract code, ensuring its accuracy and security).
Transaction costs in the context of smart contracts refer to the fees incurred during contract execution. These costs include gas fees (in Ethereum) or similar fees in other blockchain platforms. Gas is a unit used to measure the computational effort required to execute a particular operation or transaction on the blockchain.
Gas Optimization Techniques
Smart contract developers must be mindful of gas consumption, as inefficient code can lead to higher fees and reduced scalability. Let's explore some techniques to optimize gas usage and minimize transaction costs:
Minimizing Computational Complexity
One of the key factors influencing gas consumption is the computational complexity of the smart contract. By choosing efficient algorithms and data structures, developers can significantly reduce the computational overhead. Avoiding recursive functions and infinite loops is crucial, as they can lead to excessive gas consumption and even contract execution failures.
For example, suppose a decentralized application requires searching through a large dataset. In that case, developers can employ binary search algorithms instead of linear searches to reduce the number of computational steps, thereby minimizing gas costs.
Example: Using binary search instead of linear search for a sorted array to optimize gas consumption using Python.
# Linear Search (inefficient)
def linear_search (arr, target):
"""
This function performs a linear search on the given array to find the target element.
Args:
arr (list): The input array in which to search for the target.
target (int): The element to find in the array.
Returns:
int: The index of the target element if found, otherwise -1.
"""
}
for i in range (len(arr)):
if arr[i] == target:
return i
return -1
# Binary Search (efficient)
def binary_search (arr, target):
"""
This function performs a binary search on the given sorted array to find the target element.
Args:
arr (list): The sorted input array in which to search for the target.
target (int): The element to find in the array
Returns:
int: The index of the target element if found, otherwise -1.
"""
low = 0
high = len (arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
Gas-Efficient Data Storage
Data storage on the blockchain incurs significant costs, and developers must be strategic in handling data to optimize gas usage. Storing only essential data on-chain and offloading non-critical data to off-chain storage solutions (such as IPFS) can substantially reduce gas consumption.
Using data packing and bitwise operations to store multiple variables in a single storage slot is another effective optimization technique. By consolidating data, developers can save on storage costs and minimize the number of storage operations in their smart contracts.
Example: Using bitwise operations to pack multiple variables into a single storage slot.
// Gas-Efficient Data Storage Contract
contract GasEfficientDataStorage {
uint256 private packedData;
/**
* @dev Packs three uint256 variables into a single storage slot.
*
* @param a uint256: The first variable to be packed.
* @param b uint256: The second variable to be packed.
* @param c uint256: The third variable to be packed.
*/
function packData(uint256 a, uint256 b, uint256 c) public {
packedData = (a << 192) | (b << 128) | c;
}
/**
* @dev Unpacks the three uint256 variables from the storage slot.
*
* @return a uint256: The first unpacked variable.
* @return b uint256: The second unpacked variable.
* @return c uint256: The third unpacked variable.
*/
function unpackData() public view returns (uint256 a, uint256 b, uint256 c) {
a = (packedData >> 192) & uint256(0xFFFFFFFFFFFFFFFFFFFFFFFF);
b = (packedData >> 128) & uint256(0xFFFFFFFFFFFFFFFFFFFFFFFF);
c = packedData & uint256(0xFFFFFFFFFFFFFFFFFFFFFFFF);
}
}
Smart Contract Design Patterns
Leveraging established smart contract design patterns can lead to more gas-efficient and secure code. The Proxy Pattern separates the logic and data layers, enabling upgradability while minimizing gas costs. The Factory Pattern streamlines the creation of new contract instances, reducing overall gas consumption.
Example: Implementing the Proxy Pattern for upgradable smart contracts.
// Proxy Contract
contract Proxy {
address private implementation;
address private owner;
/**
* @dev Modifier to restrict function access to the contract owner only.
*/
modifier onlyOwner() {
require(msg.sender == owner, "Only the owner can perform this action");
_;
}
/**
* @dev Constructor to initialize the Proxy contract with the initial implementation and set the owner.
*
* @param initialImplementation address: The address of the initial implementation contract.
*/
constructor(address initialImplementation) {
implementation = initialImplementation;
owner = msg.sender;
}
/**
* @dev Fallback function to forward the received call to the implementation contract using delegatecall.
* It allows the Proxy contract to act as a transparent layer between the user and the implementation.
*/
fallback() external payable {
address target = implementation;
The State Machine Pattern
It is another powerful technique for organizing complex logic into states, optimizing contract execution flow, and enhancing readability and cost-effectiveness.
// State Machine Contract
contract StateMachine {
// Enum representing different states of the contract
enum State {
Created, // Contract is in the initial state after deployment
Active, // Contract is active and operational
Inactive // Contract is inactive and not performing any actions
}
// State variable to store the current state
State public currentState = State.Created;
/**
* @dev Modifier to restrict function access based on the contract's state.
* @param _state State: The required state for executing the function.
*/
modifier onlyState(State _state) {
require(currentState == _state, "Invalid contract state");
_;
}
/**
* @dev Function to transition the contract to the Active state.
* Can only be called when the contract is in the Created state.
*/
function activate() public onlyState(State.Created) {
currentState = State.Active;
// Add any logic to execute when transitioning to the Active state
}
/**
* @dev Function to transition the contract to the Inactive state.
* Can only be called when the contract is in the Active state.
*/
function deactivate() public onlyState(State.Active) {
currentState = State.Inactive;
// Add any logic to execute when transitioning to the Inactive state
}
In this example:
1. The contract uses an enum to represent different states (Created, Active, and Inactive).
2. The currentState variable tracks the current state of the contract.
3. The onlyState modifier ensures that certain functions can only be executed when the contract is in a specific state.
4. The activate function transitions the contract from the Created state to the Active state.
5. The deactivate function transitions the contract from the Active state to the Inactive state.
By using the State Machine Pattern, the contract can easily manage its different states and optimize the execution flow based on the current state. The example provided is a simplified illustration.
These examples provide a glimpse into how developers can apply the discussed optimization techniques and security best practices in actual smart contract implementations. Always keep in mind that writing secure and efficient smart contracts requires a thorough understanding of the specific platform's nuances and potential vulnerabilities.
Gas Price and Transaction Ordering
To optimize transaction costs on blockchain networks, users must consider two essential factors: gas prices and transaction ordering. These factors play a crucial role in determining the efficiency and cost-effectiveness of executing smart contracts and other blockchain transactions.
Gas Prices
In the context of blockchain, gas is a unit of measurement representing the computational resources required to execute a transaction or smart contract. Each operation within a transaction consumes a specific amount of gas, and the total gas consumed determines the transaction's cost.
Gas prices are not fixed; they are determined by the market demand and supply of computational resources.
Users can set the gas price they are willing to pay for their transactions. Miners, who validate and process transactions, prioritize those with higher gas prices since it provides them with higher rewards for their efforts.
Setting an appropriate gas price is essential to ensure the timely execution of transactions. If the gas price is too low, miners may choose to prioritize other transactions with higher gas prices, delaying the execution of low-priced transactions. On the other hand, setting a gas price too high may result in overpayment, leading to unnecessary transaction costs.
To estimate an optimal gas price, users can monitor network conditions and use tools like gas price oracles. Gas price oracles provide real-time information about prevailing gas prices on the network, allowing users to set competitive gas prices for their transactions.
Transaction Ordering
Transaction ordering refers to the sequence in which transactions are included in a block for processing by the network. The order of transactions in a block can impact gas costs and smart contract efficiency.
Resource contention can occur when multiple transactions attempt to modify the same data or smart contract state simultaneously. In such cases, miners may need to execute transactions sequentially, leading to increased gas costs and potential delays.
Optimizing transaction ordering can be achieved through various techniques such as "batching" and "transaction scheduling."
Batching
Batching involves combining multiple transactions into a single transaction. By bundling related transactions together, users can reduce the number of individual transactions, which can lead to cost savings. For example, in an Ethereum smart contract, batching multiple token transfers into a single transaction can significantly reduce gas costs.
Transaction Scheduling
Transaction scheduling involves strategically planning the execution of transactions to minimize resource contention. By considering the dependencies between transactions and organizing them accordingly, users can reduce the likelihood of conflicts and optimize gas costs.
In addition to batching and transaction scheduling, other optimization techniques, such as deploying smart contracts with fewer states or reducing unnecessary computational steps, can further enhance smart contract efficiency and minimize gas expenses.
By carefully managing gas prices and optimizing transaction ordering, users can achieve cost-efficient and timely execution of transactions on blockchain networks. This approach is especially crucial in decentralized finance (DeFi) applications and other high-frequency transaction environments, where gas costs can significantly impact the overall user experience and adoption of blockchain technology.
Example;
// SimpleToken contract representing a basic ERC20 token
contract SimpleToken {
mapping(address => uint256) public balances;
function transfer(address to, uint256 amount) public {
balances[msg.sender] -= amount;
balances[to] += amount;
}
}
// BatchingContract contract demonstrating the batching technique
contract BatchingContract {
SimpleToken public token;
constructor(address tokenAddress) {
token = SimpleToken(tokenAddress);
}
function batchTransfer(address[] memory recipients, uint256[] memory amounts) public {
require(recipients.length == amounts.length, "Invalid input arrays");
for (uint256 i = 0; i < recipients.length; i++) {
token.transfer(recipients[i], amounts[i]);
}
}
}
In this example:
1. The SimpleToken contract represents a basic ERC20 token with a transfer function for transferring tokens between addresses.
2. The BatchingContract contract takes the address of an existing SimpleToken contract as a constructor parameter.
3. The batchTransfer function in the BatchingContract contract allows users to batch multiple token transfers into a single transaction. It takes two arrays: recipients containing the addresses of recipients and amounts containing the corresponding amounts to be transferred to each recipient.
By calling the batchTransfer function with multiple recipients and amounts, users can effectively combine multiple token transfers into a single transaction, reducing the number of transactions and optimizing gas costs.
Please note that the provided example is for illustrative purposes and is simplified to demonstrate the batching technique. In real-world scenarios, batching may involve more complex logic and considerations depending on the specific use case and smart contract requirements.
Security Considerations for Smart Contracts
While optimizing transaction costs is essential, it should never come at the expense of security. Smart contracts are immutable and irreversible, making security vulnerabilities potentially catastrophic.
Example: Implementing the principle of least privilege to restrict access to specific functions.
contract LeastPrivilegeExample {
address private owner;
uint256 private secretData;
modifier onlyOwner() {
require(msg.sender == owner, "Only the owner can perform this action");
_;
}
function setSecretData(uint256 newData) public onlyOwner {
secretData = newData;
}
function getSecretData() public view onlyOwner returns (uint256) {
return secretData;
}
}
To ensure robust security, developers must adopt the following practices:
a. Comprehensive Code Audits: Thorough code reviews and audits help identify potential vulnerabilities before deployment. Security auditors use automated tools and manual inspections to uncover weaknesses and provide recommendations for improvement.
For example, let's consider a basic smart contract that handles token transfers:
}
```solidity
// BasicToken contract representing a simple ERC20 token
contract BasicToken {
mapping(address => uint256) public balances;
function transfer(address to, uint256 amount) public {
require(balances[msg.sender] >= amount, "Insufficient balance");
balances[msg.sender] -= amount;
balances[to] += amount;
}
}
```
Before deploying this contract to the blockchain, a comprehensive code audit would analyze its functionality and identify potential issues, such as integer overflows, reentrancy vulnerabilities, or lack of proper access controls.
b. Test-Driven Development (TDD): Test-Driven Development (TDD) is a development practice that enhances security and reliability. By writing test cases before actual smart contract code, developers ensure the contract behaves as intended and does not exhibit unexpected behaviour or vulnerabilities.
For instance, developers could create test cases to validate token transfers and edge cases:
```solidity
contract TestBasicToken {
BasicToken public token;
constructor() {
token = new BasicToken();
}
function testTransfer() public {
token.transfer(address(this), 100);
assert(token.balances(address(this)) == 100);
}
function testInsufficientBalance() public {
// Attempting to transfer more tokens than available balance
token.transfer(address(this), 200);
// Assertion to check that the transaction reverted due to insufficient balance
assert(!address(this).call(abi.encodeWithSignature("testInsufficientBalance()")));
}
}
```
Comprehensive unit tests, integration tests, and stress tests are essential to validate the contract's behaviour under different conditions, ensuring that it functions correctly and securely.
c. Smart Contract Best Practices: Following best practices is crucial for building secure smart contracts. The principle of least privilege, input validation, and secure data handling are core best practices that developers must adhere to.
For example, if the smart contract handles user authentication, it should follow the principle of least privilege and only request the necessary permissions from users.
Furthermore, developers must exercise caution with external calls to prevent reentrancy attacks and use reputable libraries and contracts that have undergone rigorous security audits.
d. Bug Bounties and Community Involvement: Incentivizing security researchers through bug bounties can help discover and fix vulnerabilities. Offering rewards to individuals who responsibly disclose security issues harnesses the power of the community to continually improve contract security.
Engaging with the community, seeking feedback, and collaborating on security discussions can lead to valuable insights and improvements to contract security.
By adopting these security practices and involving the community in the development process, smart contract developers can build more secure and robust applications that protect users' assets and data from potential threats and attacks.
Conclusion
Optimizing transaction costs and strengthening security is vital for the continued success and growth of smart contracts and blockchain technology. Smart contracts have shown tremendous potential to revolutionize industries by providing transparent, secure, and efficient solutions. However, to fully harness their power, developers and technical writers must be diligent in optimizing gas usage and implementing robust security measures.
By employing gas optimization techniques such as minimizing storage usage, reducing computational complexity, and utilizing efficient data structures, developers can build smart contracts that are more cost-effective for users. The careful consideration of gas prices and transaction ordering can lead to significant savings and faster execution times, enhancing the overall user experience on blockchain networks.
Furthermore, security must always remain a top priority when designing and deploying smart contracts. The immutable nature of smart contracts necessitates extra caution during development, as even the smallest vulnerabilities can have severe consequences. Adherence to best practices, thorough code audits, and continuous community involvement is indispensable for ensuring the integrity and trustworthiness of smart contracts in the decentralized world.
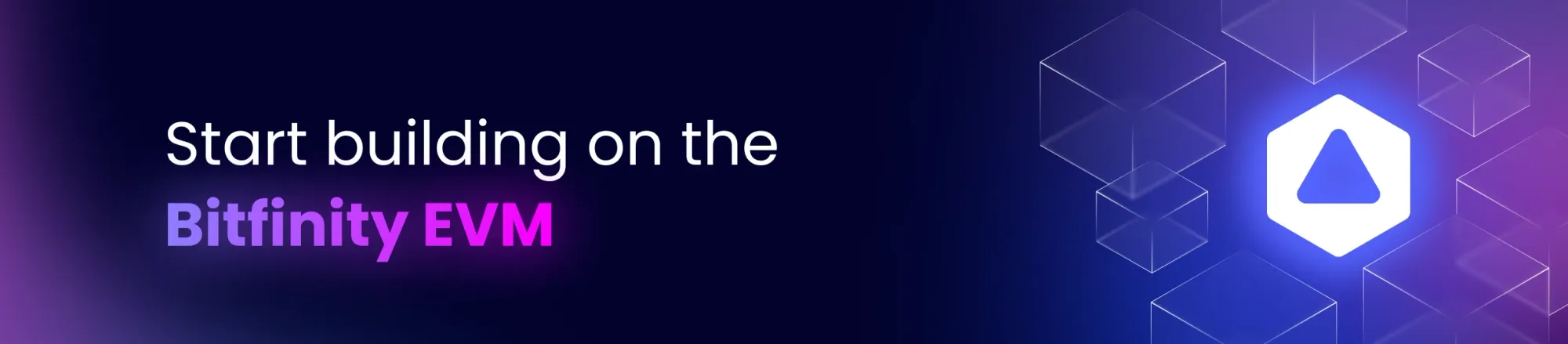
Connect with Bitfinity Network
Bitfinity Wallet | Bitfinity Network | Twitter | Telegram | Discord | Github
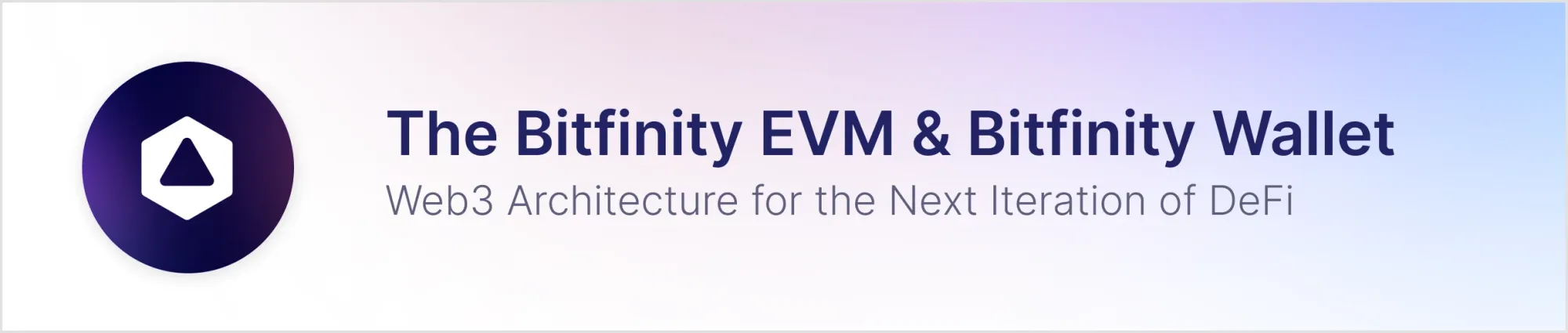
*Disclaimer: While every effort is made on this website to provide accurate information, any opinions expressed or information disseminated do not necessarily reflect the views of Bitfinity itself.
Comments ()